Angular Navbar (ナビゲーション バー) コンポーネントの概要
Ignite UI for Angular IgxNavbarComponent
は、アプリケーション内の現在位置をユーザーに通知し、ブラウザーの [戻る] ボタンのように戻る機能を提供するアプリケーション ヘッダー コンポーネントです。Navigation Bar の検索またはお気に入りなどのリンクによって、ユーザーはアプリケーションでナビゲーションをスムーズに実行できます。バーは、バーが含まれるコンテナ上に配置されます。
Angular Navbar の例
import { NgModule } from "@angular/core";
import { FormsModule } from "@angular/forms";
import { BrowserModule } from "@angular/platform-browser";
import { BrowserAnimationsModule } from "@angular/platform-browser/animations";
import { AppComponent } from "./app.component";
import {
IgxIconModule,
IgxNavbarModule
} from "igniteui-angular";
import { NavbarComponent } from "./navbar/navbar.component";
@NgModule({
bootstrap: [AppComponent],
declarations: [
AppComponent,
NavbarComponent
],
imports: [
BrowserModule,
BrowserAnimationsModule,
FormsModule,
IgxIconModule,
IgxNavbarModule
],
providers: [],
entryComponents: [],
schemas: []
})
export class AppModule {}
ts
import { Component, ViewEncapsulation } from '@angular/core';
@Component({
encapsulation: ViewEncapsulation.None,
selector: 'app-navbar',
styleUrls: ['./navbar.component.scss'],
templateUrl: './navbar.component.html'
})
export class NavbarComponent { }
ts
<article class="sample-column">
<div class="navbar-sample">
<igx-navbar title="Ignite UI for Angular">
</igx-navbar>
</div>
</article>
html
このサンプルが気に入りましたか? 完全な Ignite UI for Angularツールキットにアクセスして、すばやく独自のアプリの作成を開始します。無料でダウンロードできます。
Ignite UI for Angular Navbar を使用した作業の開始
Ignite UI for Angular Navbar コンポーネントを使用した作業を開始するには、Ignite UI for Angular をインストールする必要があります。既存の Angular アプリケーションで、以下のコマンドを入力します。
ng add igniteui-angular
cmd
Ignite UI for Angular については、「はじめに」トピックをご覧ください。
はじめに、app.module.ts ファイルに IgxNavbarModule
をインポートします。
import { IgxNavbarModule } from 'igniteui-angular';
@NgModule({
...
imports: [..., IgxNavbarModule],
...
})
export class AppModule {}
typescript
あるいは、16.0.0
以降、IgxNavbarComponent
をスタンドアロンの依存関係としてインポートすることも、IGX_NAVBAR_DIRECTIVES
トークンを使用してコンポーネントとそのすべてのサポート コンポーネントおよびディレクティブをインポートすることもできます。
import { IGX_NAVBAR_DIRECTIVES } from 'igniteui-angular';
@Component({
selector: 'app-home',
template: '<igx-navbar title="Ignite UI for Angular"></igx-navbar>',
styleUrls: ['home.component.scss'],
standalone: true,
imports: [IGX_NAVBAR_DIRECTIVES],
})
export class HomeComponent {}
typescript
Ignite UI for Angular Navbar モジュールまたはディレクティブをインポートしたので、igx-navbar
コンポーネントの使用を開始できます。
Angular Navbar の使用
コンポーネントのテンプレートで、以下のコードを追加し、タイトルの基本的な NavBar を作成します。
<igx-navbar title="Ignite UI for Angular"> </igx-navbar>
html
メニュー ボタンの追加
メニュー ボタンを追加するには、actionButtonIcon
によってアクション ボタンを表示し、以下のようにメニュー アイコンを使用します。
<igx-navbar title="Sample App" actionButtonIcon="menu" [isActionButtonVisible]="true"></igx-navbar>
html
アイコン ボタンの追加
検索、お気に入りなどのオプションを追加するには、IgxIconButton と IgxIcon モジュールを app.module.ts ファイルにインポートします。
...
import { IgxNavbarModule, IgxIconButtonDirective, IgxIconModule } from 'igniteui-angular';
@NgModule({
...
imports: [..., IgxIconButtonDirective, IgxIconModule],
})
export class AppModule {}
typescript
各オプションにアイコン ボタンを追加するためにテンプレートを更新します。
<igx-navbar title="Sample App">
<button igxIconButton="flat">
<igx-icon>search</igx-icon>
</button>
<button igxIconButton="flat">
<igx-icon>favorite</igx-icon>
</button>
<button igxIconButton="flat">
<igx-icon>more_vert</igx-icon>
</button>
</igx-navbar>
html
以下は結果です:
import { NgModule } from "@angular/core";
import { FormsModule } from "@angular/forms";
import { BrowserModule } from "@angular/platform-browser";
import { BrowserAnimationsModule } from "@angular/platform-browser/animations";
import { AppComponent } from "./app.component";
import {
IgxIconModule,
IgxNavbarModule,
IgxButtonModule
} from "igniteui-angular";
import { NavbarSample1Component } from "./navbar-sample-1/navbar-sample-1.component";
@NgModule({
bootstrap: [AppComponent],
declarations: [
AppComponent,
NavbarSample1Component
],
imports: [
BrowserModule,
BrowserAnimationsModule,
FormsModule,
IgxIconModule,
IgxNavbarModule,
IgxButtonModule
],
providers: [],
entryComponents: [],
schemas: []
})
export class AppModule {}
ts
import { Component, ViewEncapsulation } from '@angular/core';
@Component({
encapsulation: ViewEncapsulation.None,
selector: 'app-navbar-sample-1',
styleUrls: ['./navbar-sample-1.component.scss'],
templateUrl: './navbar-sample-1.component.html'
})
export class NavbarSample1Component { }
ts
<div class="sample-column">
<igx-navbar title="Sample App">
<igx-navbar-action>
<button igxButton="icon">
<igx-icon>menu</igx-icon>
</button>
</igx-navbar-action>
<button igxButton="icon">
<igx-icon>search</igx-icon>
</button>
<button igxButton="icon">
<igx-icon>favorite</igx-icon>
</button>
<button igxButton="icon">
<igx-icon>more_vert</igx-icon>
</button>
</igx-navbar>
</div>
html
カスタム動作の追加
アプリのナビゲーションでナビゲーション バーの左端にあるデフォルト アイコンではなくカスタム テンプレートを使用したい場合、igx-navbar-action
ディレクティブを使用して提供したコンテンツをレンダリングします。これには Font Awesome ホーム アイコンのボタンを使用します。
@import url("https://unpkg.com/@fortawesome/fontawesome-free-webfonts@^1.0.9/css/fontawesome.css");
@import url("https://unpkg.com/@fortawesome/fontawesome-free-webfonts@^1.0.9/css/fa-regular.css");
@import url("https://unpkg.com/@fortawesome/fontawesome-free-webfonts@^1.0.9/css/fa-solid.css");
css
<igx-navbar title="Sample App">
<igx-navbar-action>
<button igxIconButton="flat">
<igx-icon family="fa" name="fa-home"></igx-icon>
</button>
</igx-navbar-action>
<button igxIconButton="flat">
<igx-icon>search</igx-icon>
</button>
<button igxIconButton="flat">
<igx-icon>favorite</igx-icon>
</button>
<button igxIconButton="flat">
<igx-icon>more_vert</igx-icon>
</button>
</igx-navbar>
html
以下はカスタム動作ボタン アイコンをした場合の navbar の外観です。
import { NgModule } from "@angular/core";
import { FormsModule } from "@angular/forms";
import { BrowserModule } from "@angular/platform-browser";
import { BrowserAnimationsModule } from "@angular/platform-browser/animations";
import { AppComponent } from "./app.component";
import {
IgxIconModule,
IgxNavbarModule,
IgxButtonModule
} from "igniteui-angular";
import { NavbarSample2Component } from "./navbar-sample-2/navbar-sample-2.component";
@NgModule({
bootstrap: [AppComponent],
declarations: [
AppComponent,
NavbarSample2Component,
IgxButtonModule
],
imports: [
BrowserModule,
BrowserAnimationsModule,
FormsModule,
IgxIconModule,
IgxNavbarModule
],
providers: [],
entryComponents: [],
schemas: []
})
export class AppModule {}
ts
import { Component, ViewEncapsulation } from '@angular/core';
@Component({
encapsulation: ViewEncapsulation.None,
selector: 'app-navbar-sample-2',
styleUrls: ['./navbar-sample-2.component.scss'],
templateUrl: './navbar-sample-2.component.html'
})
export class NavbarSample2Component { }
ts
<div class="sample-column">
<igx-navbar title="Sample App">
<igx-navbar-action>
<button igxButton="icon">
<igx-icon family="fa" name="fa-home"></igx-icon>
</button>
</igx-navbar-action>
<button igxButton="icon">
<igx-icon>search</igx-icon>
</button>
<button igxButton="icon">
<igx-icon>favorite</igx-icon>
</button>
<button igxButton="icon">
<igx-icon>more_vert</igx-icon>
</button>
</igx-navbar>
</div>
html
@import url("https://unpkg.com/@fortawesome/fontawesome-free-webfonts@^1.0.9/css/fontawesome.css");
@import url("https://unpkg.com/@fortawesome/fontawesome-free-webfonts@^1.0.9/css/fa-regular.css");
@import url("https://unpkg.com/@fortawesome/fontawesome-free-webfonts@^1.0.9/css/fa-solid.css");
scss
ナビゲーション アイコを追加
戻るためのアイコンが付いたナビゲーション バーを作成する場合は、次の手順を実行します。まず、actionButtonIcon
プロパティを使用して、Material フォントセットから適切なアイコンを選択できます。次に、以前にアクセスしたページに戻るかどうかを確認し、その結果を isActionButtonVisible
プロパティに渡します。最後の手順は、戻るためのメソッドを作成し、action
プロパティにフックすることです。
<igx-navbar
title="Ignite UI for Angular"
actionButtonIcon="arrow_back"
[isActionButtonVisible]="canGoBack()"
(action)="navigateBack()"
>
</igx-navbar>
html
export class NavbarSample3Component {
constructor(private _location: Location) {}
public ngOnInit() {}
public navigateBack() {
this._location.back();
}
public canGoBack() {
return window.history.length > 0;
}
}
typescript
サンプルが正しく構成された場合、ブラウザーで以下が表示されます。
import { NgModule } from "@angular/core";
import { FormsModule } from "@angular/forms";
import { BrowserModule } from "@angular/platform-browser";
import { BrowserAnimationsModule } from "@angular/platform-browser/animations";
import { AppComponent } from "./app.component";
import {
IgxIconModule,
IgxNavbarModule
} from "igniteui-angular";
import { NavbarSample3Component } from "./navbar-sample-3/navbar-sample-3.component";
@NgModule({
bootstrap: [AppComponent],
declarations: [
AppComponent,
NavbarSample3Component
],
imports: [
BrowserModule,
BrowserAnimationsModule,
FormsModule,
IgxIconModule,
IgxNavbarModule
],
providers: [],
entryComponents: [],
schemas: []
})
export class AppModule {}
ts
import { Location, LocationStrategy, PathLocationStrategy } from '@angular/common';
import { Component } from '@angular/core';
@Component({
providers: [Location, { provide: LocationStrategy, useClass: PathLocationStrategy }],
selector: 'app-navbar',
styleUrls: ['./navbar-sample-3.component.scss'],
templateUrl: './navbar-sample-3.component.html'
})
export class NavbarSample3Component {
constructor(private _location: Location) { }
public navigateBack() {
this._location.back();
}
public canGoBack() {
return window.history.length > 0;
}
}
ts
<div class="sample-column">
<igx-navbar title="Ignite UI for Angular" actionButtonIcon="arrow_back" [isActionButtonVisible]="canGoBack()"
(action)="navigateBack()">
</igx-navbar>
</div>
html
カスタムのタイトルを追加する
Navbar のタイトルにカスタム コンテンツを提供する場合は、igx-navbar-title
または igxNavbarTitle
ディレクティブを使用ます。これらは、title
入力プロパティによって提供されるデフォルトの navbar のタイトルを置き換えます。以下のサンプルには、画像付きのリンクを含むカスタム タイトルがあります。
<div class="sample-column">
<igx-navbar>
<igx-navbar-action>
<button igxIconButton="flat">
<igx-icon>menu</igx-icon>
</button>
</igx-navbar-action>
<div igxNavbarTitle>
<a href="https://www.infragistics.com/products/ignite-ui-angular" target="_blank">
<img
src="https://static.infragistics.com/marketing/Website/products/ignite-ui-landing/ignite-ui-logo.svg"
width="120px"
height="50px"
alt
style="margin-top: 7px;"
/>
</a>
</div>
<button igxIconButton="flat">
<igx-icon>search</igx-icon>
</button>
<button igxIconButton="flat">
<igx-icon>favorite</igx-icon>
</button>
<button igxIconButton="flat">
<igx-icon>more_vert</igx-icon>
</button>
</igx-navbar>
</div>
html
import { NgModule } from "@angular/core";
import { FormsModule } from "@angular/forms";
import { BrowserModule } from "@angular/platform-browser";
import { BrowserAnimationsModule } from "@angular/platform-browser/animations";
import { AppComponent } from "./app.component";
import {
IgxIconModule,
IgxNavbarModule,
IgxButtonModule
} from "igniteui-angular";
import { NavbarCustomTitleComponent } from "./navbar-custom-title/navbar-custom-title.component";
@NgModule({
bootstrap: [AppComponent],
declarations: [
AppComponent,
NavbarCustomTitleComponent
],
imports: [
BrowserModule,
BrowserAnimationsModule,
FormsModule,
IgxIconModule,
IgxNavbarModule,
IgxButtonModule
],
providers: [],
entryComponents: [],
schemas: []
})
export class AppModule {}
ts
import { Component } from '@angular/core';
@Component({
selector: 'app-navbar-custom-title',
templateUrl: './navbar-custom-title.component.html',
styleUrls: ['./navbar-custom-title.component.scss']
})
export class NavbarCustomTitleComponent { }
ts
<div class="sample-column">
<igx-navbar>
<igx-navbar-action>
<button igxButton="icon">
<igx-icon>menu</igx-icon>
</button>
</igx-navbar-action>
<div igxNavbarTitle>
<a href="https://www.infragistics.com/products/ignite-ui-angular" target="_blank">
<img src="https://static.infragistics.com/marketing/Website/products/ignite-ui-landing/ignite-ui-logo.svg"
width="120px" height="50px" alt="" style="margin-top: 7px;">
</a>
</div>
<button igxButton="icon">
<igx-icon>search</igx-icon>
</button>
<button igxButton="icon">
<igx-icon>favorite</igx-icon>
</button>
<button igxButton="icon">
<igx-icon>more_vert</igx-icon>
</button>
</igx-navbar>
</div>
html
:host ::ng-deep {
.igx-navbar {
padding: 0 16px;
}
}
scss
スタイル設定
ページネーターのスタイル設定を始めるには、すべてのテーマ関数とコンポーネントミックスインが存在する index
ファイルをインポートする必要があります。
@use "igniteui-angular/theming" as *;
scss
最も簡単な方法は、navbar-theme
を拡張する新しいテーマを作成し、$text-color
、$background
、$idle-icon-color
、$hover-icon-color
パラメーターを受け取る方法です。
$custom-navbar-theme: navbar-theme(
$text-color: #151515,
$background: #dedede,
$idle-icon-color: #151515,
$hover-icon-color: #8c8c8c,
);
scss
上記のようにカラーの値をハードコーディングする代わりに、palette
および color
関数を使用してカラーに関してより高い柔軟性を実現することができます。使い方の詳細についてはパレット
のトピックをご覧ください。
最後に、新しく作成されたテーマを css-vars
ミックスインに渡します。
@include css-vars($custom-navbar-theme);
scss
デモ
import { NgModule } from "@angular/core";
import { FormsModule } from "@angular/forms";
import { BrowserModule } from "@angular/platform-browser";
import { BrowserAnimationsModule } from "@angular/platform-browser/animations";
import { AppComponent } from "./app.component";
import {
IgxIconModule,
IgxNavbarModule,
IgxButtonModule
} from "igniteui-angular";
import { NavbarStyleComponent } from "./navbar-style/navbar-style.component";
@NgModule({
bootstrap: [AppComponent],
declarations: [
AppComponent,
NavbarStyleComponent
],
imports: [
BrowserModule,
BrowserAnimationsModule,
FormsModule,
IgxIconModule,
IgxNavbarModule,
IgxButtonModule
],
providers: [],
entryComponents: [],
schemas: []
})
export class AppModule {}
ts
import { Component } from '@angular/core';
@Component({
selector: 'app-navbar-style',
styleUrls: ['./navbar-style.component.scss'],
templateUrl: './navbar-style.component.html'
})
export class NavbarStyleComponent { }
ts
<div class="sample-column">
<igx-navbar title="Sample App">
<igx-navbar-action>
<button igxButton="icon">
<igx-icon>menu</igx-icon>
</button>
</igx-navbar-action>
<button igxButton="icon">
<igx-icon>search</igx-icon>
</button>
<button igxButton="icon">
<igx-icon>favorite</igx-icon>
</button>
<button igxButton="icon">
<igx-icon>more_vert</igx-icon>
</button>
</igx-navbar>
</div>
html
@use '../../variables' as *;
$custom-navbar-theme: navbar-theme(
$text-color: #151515,
$background: #dedede,
$idle-icon-color: #151515,
$hover-icon-color: #8c8c8c
);
:host {
@include css-vars($custom-navbar-theme);
}
scss
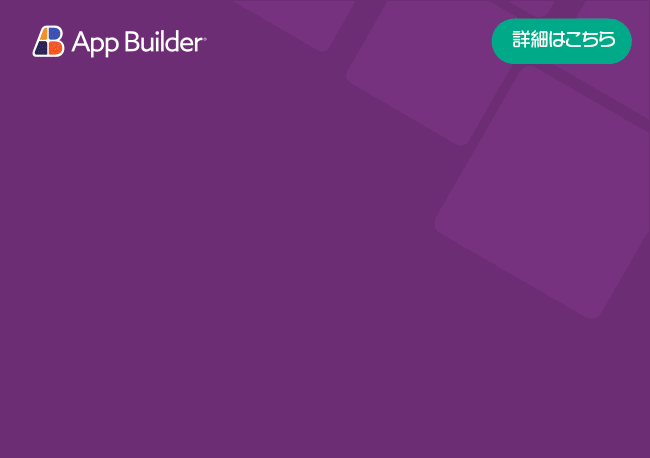
API リファレンス
その他のコンポーネントおよびディレクティブ (またはそのいずれか) で使用した API:
テーマの依存関係
その他のリソース
コミュニティに参加して新しいアイデアをご提案ください。