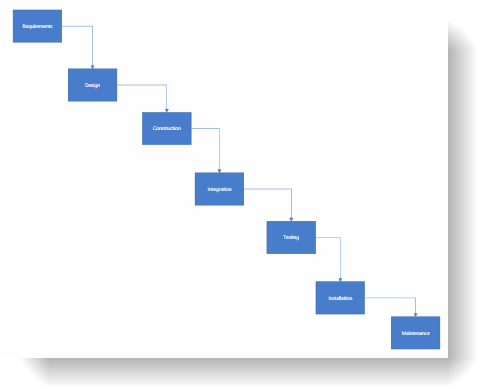
以下の手順では、入力データ オブジェクトがノードおよび接続を表すデータに xamDiagram をバインドする方法を説明します。ノード オブジェクトはそれぞれ一意の識別子を表す object
型のプロパティを持ち、接続オブジェクトはそれぞれ開始ノードと終了ノードを表す 2 つのノード オブジェクトの識別子を保持する 2 つのプロパティを持ちます。
以下のスクリーンショットは結果のプレビューです。
この手順を実行するには、以下が必要です。
ページに追加される空の xamDiagram を持つ WPF アプリケーション
ForceDirectedGraphDiagramLayout のインスタンスに設定される xamDiagram の Layout プロパティ
以下はプロセスの概要です。
ItemsSource と ConnectionSource の設定*
ノード定義の作成*
接続定義の作成*
以下の手順は、キーを持つノードおよび接続データに xamDiagram をバインドして、Waterflow ソフトウェアの開発プロセスのダイアグラムを作成する方法を示します。
サンプル ノードのデータ クラスを追加します。
以下の Activity
クラスをコード ビハインドに追加します。Activity
はノード オブジェクトを表し、Key
と呼ばれる単一の文字列プロパティを持ちます。
C# の場合:
public class Activity : INotifyPropertyChanged
{
public Activity(string name) { Key = name; }
private string _key;
public string Key {
get { return _key; }
set
{
_key = value;
OnPropertyChanged();
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = "")
{
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
VB の場合:
Public Class Activity
Implements INotifyPropertyChanged
Public Sub New(name As String)
Key = name
End Sub
Private _key As String
Public Property Key() As String
Get
Return _key
End Get
Set
_key = value
OnPropertyChanged()
End Set
End Property
Public Event PropertyChanged As PropertyChangedEventHandler
Protected Sub OnPropertyChanged( Optional propertyName As String = "")
RaiseEvent PropertyChanged(Me, New PropertyChangedEventArgs(propertyName))
End Sub
End Class
サンプルの接続クラスを追加します。
以下の ActivityRelation
クラスをコード ビハインドに追加します。ActivityRelation
クラスは、2 つの Activity
インスタンスの間の指示された接続を表します。開始アクティビティと終了アクティビティのキーに設定された 2 つの文字列プロパティを持ちます。
C# の場合:
public class ActivityRelation : INotifyPropertyChanged
{
public ActivityRelation(string from, string to)
{
StartKey = from;
EndKey = to;
}
private string _startKey;
public string StartKey
{
get { return _startKey; }
set
{
_startKey = value;
OnPropertyChanged();
}
}
private string _endKey;
public string EndKey
{
get { return _endKey; }
set
{
_endKey = value;
OnPropertyChanged();
}
}
public override string ToString()
{
return "";
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = "")
{
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
VB の場合:
Public Class ActivityRelation
Implements INotifyPropertyChanged
Public Sub New(from As String, [to] As String)
StartKey = from
EndKey = [to]
End Sub
Private _startKey As String
Public Property StartKey() As String
Get
Return _startKey
End Get
Set
_startKey = value
OnPropertyChanged()
End Set
End Property
Private _endKey As String
Public Property EndKey() As String
Get
Return _endKey
End Get
Set
_endKey = value
OnPropertyChanged()
End Set
End Property
Public Overrides Function ToString() As String
Return ""
End Function
Public Event PropertyChanged As PropertyChangedEventHandler
Protected Sub OnPropertyChanged( Optional propertyName As String = "")
RaiseEvent PropertyChanged(Me, New PropertyChangedEventArgs(propertyName))
End Sub
End Class
データ項目を追加します。
以下の WaterFlowViewModel
クラスを追加すると、ノードおよび接続のための 2 つの リスト プロパティが表示され、サンプル データが自動的に事前設定されます。
C# の場合:
public class WaterFlowViewModel : INotifyPropertyChanged
{
public WaterFlowViewModel()
{
Activities = new ObservableCollection<Activity>()
{
new Activity("Requirements"),
new Activity("Design"),
new Activity("Construction"),
new Activity("Integration"),
new Activity("Testing"),
new Activity("Installation"),
new Activity("Maintenance")
};
Relations = new ObservableCollection<ActivityRelation>()
{
new ActivityRelation("Requirements", "Design"),
new ActivityRelation("Design", "Construction"),
new ActivityRelation("Construction", "Integration"),
new ActivityRelation("Integration", "Testing"),
new ActivityRelation("Testing", "Installation"),
new ActivityRelation("Installation", "Maintenance"),
};
}
private IList<Activity> _activities;
public IList<Activity> Activities
{
get { return _activities; }
set
{
_activities = value;
OnPropertyChanged();
}
}
private IList<ActivityRelation> _relations;
public IList<ActivityRelation> Relations
{
get { return _relations; }
set
{
_relations = value;
OnPropertyChanged();
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = "")
{
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
VB の場合:
Public Class WaterFlowViewModel
Implements INotifyPropertyChanged
Public Sub New()
Activities = New ObservableCollection(Of Activity)() From { _
New Activity("Requirements"), _
New Activity("Design"), _
New Activity("Construction"), _
New Activity("Integration"), _
New Activity("Testing"), _
New Activity("Installation"), _
New Activity("Maintenance") _
}
Relations = New ObservableCollection(Of ActivityRelation)() From { _
New ActivityRelation("Requirements", "Design"), _
New ActivityRelation("Design", "Construction"), _
New ActivityRelation("Construction", "Integration"), _
New ActivityRelation("Integration", "Testing"), _
New ActivityRelation("Testing", "Installation"), _
New ActivityRelation("Installation", "Maintenance") _
}
End Sub
Private _activities As IList(Of Activity)
Public Property Activities() As IList(Of Activity)
Get
Return _activities
End Get
Set(value As IList(Of Activity))
_activities = value
OnPropertyChanged()
End Set
End Property
Private _relations As IList(Of ActivityRelation)
Public Property Relations() As IList(Of ActivityRelation)
Get
Return _relations
End Get
Set(value As IList(Of ActivityRelation))
_relations = value
OnPropertyChanged()
End Set
End Property
Public Event PropertyChanged As PropertyChangedEventHandler Implements INotifyPropertyChanged.PropertyChanged
Protected Sub OnPropertyChanged(Optional propertyName As String = "")
RaiseEvent PropertyChanged(Me, New PropertyChangedEventArgs(propertyName))
End Sub
End Class
XamDiagram の DataContext を設定します。
ダイアグラムの DataContext
プロパティを WaterFlowViewModel
クラスのインスタンスに設定します。
XAML の場合:
<ig:XamDiagram>
<ig:XamDiagram.DataContext>
<local:WaterFlowViewModel/>
</ig:XamDiagram.DataContext>
</ig:XamDiagram>
ItemsSource プロパティを設定します。
ItemsSource を WaterFlowViewModel
のデータ コンテキストの Articles
プロパティにバインドします。
XAML の場合:
ItemsSource="{Binding Activities}"
ConnectionsSource プロパティを設定します。
ConnectionsSource を WaterFlowViewModel
のデータ コンテキストの Relations
プロパティにバインドします。
XAML の場合:
ConnectionsSource="{Binding Relations}"
通常、 ItemsSource および NodeDefinition の各データ タイプが xamDiagram に追加されます。1 つ以上のタイプに継承関係がある場合、最初に、最も具体的なタイプを指定します。xamDiagram は、各データ項目のタイプをノード定義の TargetType に一致させようとします。その TargetType が IsAssignableFrom
への呼び出しから true を返す、最初のノード定義が選択されます。これは、ノード定義の TargetType が完全に一致する場合、またはデータ項目のタイプの親タイプである場合に該当し、そうでない場合はノード定義が選択されます。
キーを持つデータ バインディングを使用するには、ノード オブジェクトが DiagramNode クラスの Key プロパティの有効な値がある文字列プロパティを持っている必要があります。
Activity
クラスに NodeDefinition を作成して、NodeDefinitions コレクションに追加します。
NodeDefinition の TargetType を Activity
型に設定します。
KeyMemberPath を Key に設定します。これは作成された DiagramNode インスタンスの Name プロパティを Key プロティの値に設定します。
DisplayMemberPath を Key に設定します。
DisplayMemberPath を定義しないで、NodeStyle を介してカスタム DisplayTemplate を設定しないと ToString
メソッドはノードのコンテンツとして表示されます。
(オプション) NodeStyle を設定します。
NodeStyle プロパティを使用すると、ノード定義によって一致するすべての DiagramNode
オブジェクトに適用するスタイルを設定できます。これにより、特定のデータ タイプに対して作成されたノードのカスタマイズが容易になります。
XAML の場合:
<ig:XamDiagram.NodeDefinitions>
<ig:NodeDefinition
TargetType="local:Activity"
KeyMemberPath="Key"
DisplayMemberPath="Key">
<ig:NodeDefinition.NodeStyle>
<Style TargetType="ig:DiagramNode">
<Setter Property="ConnectionPoints">
<Setter.Value>
<ig:DiagramConnectionPointCollection>
<ig:DiagramConnectionPoint Name="Top" Position="0.5, 0"/>
<ig:DiagramConnectionPoint Name="Right" Position="1, 0.5"/>
</ig:DiagramConnectionPointCollection>
</Setter.Value>
</Setter>
</Style>
</ig:NodeDefinition.NodeStyle>
</ig:NodeDefinition>
</ig:XamDiagram.NodeDefinitions>
個別の接続データ オブジェクトを指定する場合は、ダイアグラムの ConnectionDefinitions の ConnectionSourceDefinition のインスタンスを使用します。このタイプの接続定義では、定義の TargetType に一致する各データ項目に対して作成する接続を記述します。このような接続に StartNodeKey プロパティと EndNodeKey プロパティを正しく事前設定するには、接続定義の StartNodeKeyMemberPath プロパティおよび EndNodeKeyMemberPath プロパティを、開始 / 終了ノードのデータ オブジェクトの参照を保持するプロパティ名に設定することが必要です。
ConnectionSourceDefinition を作成して、ConnectionDefinitions コレクションに追加します。
TargetType を ActivityRelation
型に設定します。
StartNodeKeyMemberPath を "StartKey" に設定します。 EndNodeKeyMemberPath を "EndKey" に設定します。
(オプション) ConnectionStyle を設定します。
DiagramConnection を対象とするスタイルに ConnectionStyle プロパティを設定すると、特定のデータ タイプに対して作成される接続をカスタマイズできます。DisplayTemplate プロパティの設定を指定し、データ オブジェクトが作成される DataContext
を設定するテンプレートを適用できます。
XAML の場合:
<ig:XamDiagram.ConnectionDefinitions>
<ig:ConnectionSourceDefinition
TargetType="local:ActivityRelation"
StartNodeKeyMemberPath="StartKey"
EndNodeKeyMemberPath="EndKey"/>
</ig:XamDiagram.ConnectionDefinitions>
以下は、この手順の完全なコードです。
XAML の場合:
<UserControl x:Class="DiagramDocumentationSamples.NodesConnectionsKeysData"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:ig="http://schemas.infragistics.com/xaml"
xmlns:local="clr-namespace:DiagramDocumentationSamples"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="300">
<ig:XamDiagram ConnectionsSource="{Binding Relations}" ItemsSource="{Binding Activities}">
<ig:XamDiagram.DataContext>
<local:WaterFlowViewModel/>
</ig:XamDiagram.DataContext>
<ig:XamDiagram.NodeDefinitions>
<ig:NodeDefinition
TargetType="local:Activity"
KeyMemberPath="Key"
DisplayMemberPath="Key">
<ig:NodeDefinition.NodeStyle>
<Style TargetType="ig:DiagramNode">
<Setter Property="ConnectionPoints">
<Setter.Value>
<ig:DiagramConnectionPointCollection>
<ig:DiagramConnectionPoint Name="Top" Position="0.5, 0"/>
<ig:DiagramConnectionPoint Name="Right" Position="1, 0.5"/>
</ig:DiagramConnectionPointCollection>
</Setter.Value>
</Setter>
</Style>
</ig:NodeDefinition.NodeStyle>
</ig:NodeDefinition>
</ig:XamDiagram.NodeDefinitions>
<ig:XamDiagram.ConnectionDefinitions>
<ig:ConnectionSourceDefinition
TargetType="local:ActivityRelation"
StartNodeKeyMemberPath="StartKey"
EndNodeKeyMemberPath="EndKey"/>
</ig:XamDiagram.ConnectionDefinitions>
<ig:XamDiagram.Layout>
<ig:ForceDirectedGraphDiagramLayout/>
</ig:XamDiagram.Layout>
</ig:XamDiagram>
</UserControl>
C# の場合:
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Runtime.CompilerServices;
using System.Windows.Controls;
namespace DiagramDocumentationSamples
{
public partial class NodesConnectionsKeysData : UserControl
{
public NodesConnectionsKeysData()
{
InitializeComponent();
}
}
public class WaterFlowViewModel : INotifyPropertyChanged
{
public WaterFlowViewModel()
{
Activities = new ObservableCollection<Activity>()
{
new Activity("Requirements"),
new Activity("Design"),
new Activity("Construction"),
new Activity("Integration"),
new Activity("Testing"),
new Activity("Installation"),
new Activity("Maintenance")
};
Relations = new ObservableCollection<ActivityRelation>()
{
new ActivityRelation("Requirements", "Design"),
new ActivityRelation("Design", "Construction"),
new ActivityRelation("Construction", "Integration"),
new ActivityRelation("Integration", "Testing"),
new ActivityRelation("Testing", "Installation"),
new ActivityRelation("Installation", "Maintenance"),
};
}
private IList<Activity> _activities;
public IList<Activity> Activities
{
get { return _activities; }
set
{
_activities = value;
OnPropertyChanged();
}
}
private IList<ActivityRelation> _relations;
public IList<ActivityRelation> Relations
{
get { return _relations; }
set
{
_relations = value;
OnPropertyChanged();
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = "")
{
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
public class Activity : INotifyPropertyChanged
{
public Activity(string name) { Key = name; }
private string _key;
public string Key
{
get { return _key; }
set
{
_key = value;
OnPropertyChanged();
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = "")
{
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
public class ActivityRelation : INotifyPropertyChanged
{
public ActivityRelation(string from, string to)
{
StartKey = from;
EndKey = to;
}
private string _startKey;
public string StartKey
{
get { return _startKey; }
set
{
_startKey = value;
OnPropertyChanged();
}
}
private string _endKey;
public string EndKey
{
get { return _endKey; }
set
{
_endKey = value;
OnPropertyChanged();
}
}
public override string ToString()
{
return "";
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = "")
{
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
}
VB の場合:
Imports System.Collections.Generic
Imports System.Collections.ObjectModel
Imports System.ComponentModel
Imports System.Runtime.CompilerServices
Imports System.Windows.Controls
Namespace DiagramDocumentationSamples
Public Partial Class NodesConnectionsKeysData
Inherits UserControl
Public Sub New()
InitializeComponent()
End Sub
End Class
Public Class WaterFlowViewModel
Implements INotifyPropertyChanged
Public Sub New()
Activities = New ObservableCollection(Of Activity)() From { _
New Activity("Requirements"), _
New Activity("Design"), _
New Activity("Construction"), _
New Activity("Integration"), _
New Activity("Testing"), _
New Activity("Installation"), _
New Activity("Maintenance") _
}
Relations = New ObservableCollection(Of ActivityRelation)() From { _
New ActivityRelation("Requirements", "Design"), _
New ActivityRelation("Design", "Construction"), _
New ActivityRelation("Construction", "Integration"), _
New ActivityRelation("Integration", "Testing"), _
New ActivityRelation("Testing", "Installation"), _
New ActivityRelation("Installation", "Maintenance") _
}
End Sub
Private _activities As IList(Of Activity)
Public Property Activities() As IList(Of Activity)
Get
Return _activities
End Get
Set(value As IList(Of Activity))
_activities = value
OnPropertyChanged()
End Set
End Property
Private _relations As IList(Of ActivityRelation)
Public Property Relations() As IList(Of ActivityRelation)
Get
Return _relations
End Get
Set(value As IList(Of ActivityRelation))
_relations = value
OnPropertyChanged()
End Set
End Property
Public Event PropertyChanged As PropertyChangedEventHandler Implements INotifyPropertyChanged.PropertyChanged
Protected Sub OnPropertyChanged(Optional propertyName As String = "")
RaiseEvent PropertyChanged(Me, New PropertyChangedEventArgs(propertyName))
End Sub
End Class
Public Class Activity
Implements INotifyPropertyChanged
Public Sub New(name As String)
Key = name
End Sub
Private _key As String
Public Property Key() As String
Get
Return _key
End Get
Set(value As String)
_key = value
OnPropertyChanged()
End Set
End Property
Public Event PropertyChanged As PropertyChangedEventHandler Implements INotifyPropertyChanged.PropertyChanged
Protected Sub OnPropertyChanged(Optional propertyName As String = "")
RaiseEvent PropertyChanged(Me, New PropertyChangedEventArgs(propertyName))
End Sub
End Class
Public Class ActivityRelation
Implements INotifyPropertyChanged
Public Sub New(from As String, [to] As String)
StartKey = from
EndKey = [to]
End Sub
Private _startKey As String
Public Property StartKey() As String
Get
Return _startKey
End Get
Set(value As String)
_startKey = value
OnPropertyChanged()
End Set
End Property
Private _endKey As String
Public Property EndKey() As String
Get
Return _endKey
End Get
Set(value As String)
_endKey = value
OnPropertyChanged()
End Set
End Property
Public Overrides Function ToString() As String
Return ""
End Function
Public Event PropertyChanged As PropertyChangedEventHandler Implements INotifyPropertyChanged.PropertyChanged
Protected Sub OnPropertyChanged(Optional propertyName As String = "")
RaiseEvent PropertyChanged(Me, New PropertyChangedEventArgs(propertyName))
End Sub
End Class
End Namespace
このトピックの追加情報については、以下のトピックも合わせてご参照ください。