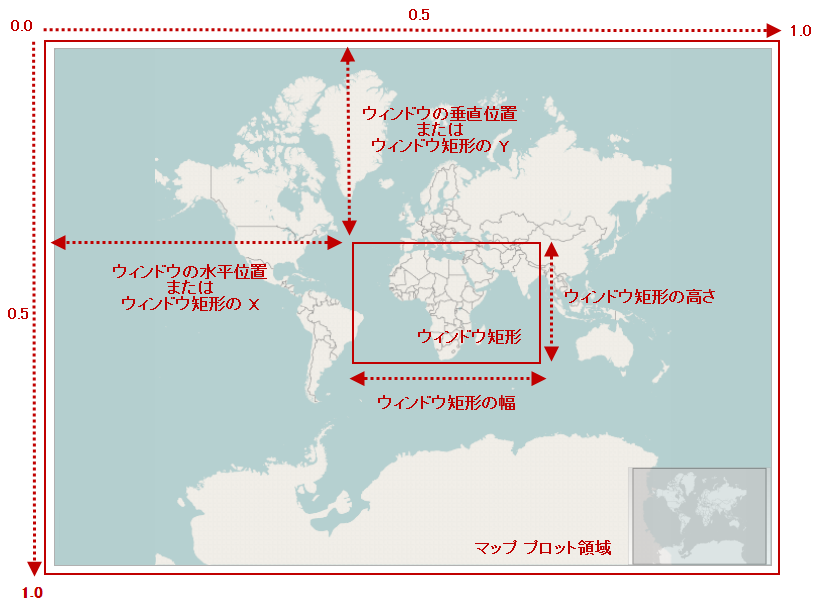
このトピックは、コードを使用して、 UltraGeographicMap™ コントロールのマップ コンテンツをナビゲートする情報を提供します。
以下の表に、このトピックを理解するための前提条件として求められるトピックをリストします。
このトピックには次のセクションがあります。
UltraGeographicMap コントロールを使用して 2 つの方法でナビゲートをすることができ、コードを使用してマップ コンテンツをナビゲートします。最初はウィンドウ ナビゲーション システムを使用する方法で、2 番目はワールド ナビゲーション システムを使用する方法です。
ウィンドウ ナビゲーション システム
ワールド ナビゲーション システム
UltraGeographicMap コントロールは、値を変換するメソッドを提供することで、両方のナビゲーション システムに対応します。これらの方法については、このトピックの実例のセクションをごらんください。
ウィンドウ ナビゲーション システムは、 WindowRect の位置とサイズ(ウィンドウ座標のマップ ビュー)を設定するためのプロパティで構成されます。このナビゲーション システムでは、値はマップ ウィンドウ ビューのプロパティで 0 および 1 に設定できます。
次のプレビュー画像は UltraGeographicMap コントロールで WindowRect の位置やサイズが強調表示され、マップ コンテンツのある特定の地域 (アフリカ大陸やヨーロッパ大陸など) にズームしています。
以下の表では、UltraGeographicMap コントロールのマップ ウィンドウの位置とサイズを設定するプロパティについて簡単に説明します。
ワールド ナビゲーション システムは、WorldRect の位置とサイズを設定するためのプロパティで構成されます(地理的座標のマップ ビュー)。このナビゲーション システムでは、WorldRect の経度プロパティでは -180~180 の値、 WorldRect ビューの緯度プロパティでは -85~85 の値に設定できます。
次のプレビュー画像は UltraGeographicMap コントロールで WorldRect の位置やサイズが強調表示され、マップ コンテンツのある特定の地域 (アフリカ大陸やヨーロッパ大陸など) にズームしています。
以下の表では、UltraGeographicMap コントロールのマップ ウィンドウの位置とサイズを設定するプロパティについて簡単に説明します。
以下の表で、UltraGeographicMap コントロールでサポートされているコード ナビゲーション機能について簡単に説明します。
C# の場合:
// 0.1 の係数で地図の中心にズームインします
var widthScale = (0.1 * this.GeoMap.WindowRect.Width);
var heightScale = (0.1 * this.GeoMap.WindowRect.Height);
var x = this.GeoMap.WindowRect.X + (widthScale / 2);
var y = this.GeoMap.WindowRect.Y + (heightScale / 2);
var w = this.GeoMap.WindowRect.Width - widthScale;
var h = this.GeoMap.WindowRect.Height - heightScale;
this.GeoMap.WindowRect = new Rectangle(x, y, w, h);
Visual Basic の場合:
' 0.1 の係数で地図の中心にズームインします
Dim widthScale = (0.1 * Me.GeoMap.WindowRect.Width)
Dim heightScale = (0.1 * Me.GeoMap.WindowRect.Height)
Dim x = Me.GeoMap.WindowRect.X + (widthScale / 2)
Dim y = Me.GeoMap.WindowRect.Y + (heightScale / 2)
Dim w = Me.GeoMap.WindowRect.Width - widthScale
Dim h = Me.GeoMap.WindowRect.Height - heightScale
Me.GeoMap.WindowRect = New Rectangle(x, y, w, h)
C# の場合:
// 0.1 の係数でズームアウトします
var widthScale = (0.1 * this.GeoMap.WindowRect.Width);
var heightScale = (0.1 * this.GeoMap.WindowRect.Height);
var x = this.GeoMap.WindowRect.X - (widthScale / 2);
var y = this.GeoMap.WindowRect.Y - (heightScale / 2);
var w = this.GeoMap.WindowRect.Width + widthScale;
var h = this.GeoMap.WindowRect.Height + heightScale;
this.GeoMap.WindowRect = new Rectangle(x, y, w, h);
Visual Basic の場合:
' 0.1 の係数でズームアウトします
Dim widthScale = (0.1 * Me.GeoMap.WindowRect.Width)
Dim heightScale = (0.1 * Me.GeoMap.WindowRect.Height)
Dim x = Me.GeoMap.WindowRect.X - (widthScale / 2)
Dim y = Me.GeoMap.WindowRect.Y - (heightScale / 2)
Dim w = Me.GeoMap.WindowRect.Width + widthScale
Dim h = Me.GeoMap.WindowRect.Height + heightScale
Me.GeoMap.WindowRect = New Rectangle(x, y, w, h)
C# の場合:
// マップ ウィンドウの座標を使用してマップ エリアへズームします
this.GeoMap.WindowRect = new Rectangle(0.2, 0.3, 0.6, 0.4);
Visual Basic の場合:
' マップ ウィンドウの座標を使用してマップ エリアへズームします
Me.GeoMap.WindowRect = New Rectangle(0.2, 0.3, 0.6, 0.4)
C# の場合:
// マップ ウィンドウの地理的座標を使用してマップ エリアへズームします
var geoRegion = new Rectangle(-30, -40, 120, 80);
this.GeoMap.WindowRect = this.GeoMap.GetZoomFromGeographic(geoRegion);
Visual Basic の場合:
' マップ ウィンドウの地理的座標を使用してマップ エリアへズームします
Dim geoRegion = New Rectangle(-30, -40, 120, 80)
Me.GeoMap.WindowRect = Me.GeoMap.GetZoomFromGeographic(geoRegion)
C# の場合:
// マップ ウィンドウ座標を使用してマップ コンテンツの表示領域を最大表示に合わせます
this.GeoMap.WindowRect = new Rectangle(0.0, 0.0, 1.0, 1.0);
// 世界地図座標を使用してマップ コンテンツの表示領域を最大表示に合わせます
var geoRegion = new Rectangle(-180, -75, 360, 150);
this.GeoMap.WindowRect = this.GeoMap.GetZoomFromGeographic(geoRegion);
Visual Basic の場合:
' マップ ウィンドウ座標を使用してマップ コンテンツの表示領域を最大表示に合わせます
Me.GeoMap.WindowRect = New Rectangle(0.0, 0.0, 1.0, 1.0)
' 世界地図座標を使用してマップ コンテンツの表示領域を最大表示に合わせます
Dim geoRegion = New Rectangle(-180, -75, 360, 150)
Me.GeoMap.WindowRect = Me.GeoMap.GetZoomFromGeographic(geoRegion)
C# の場合:
// マップ コンテンツを地理的領域にバインドおよび制限します
this.GeoMap.WorldRect = new Rectangle(-30, -40, 120, 80);
Visual Basic の場合:
' マップ コンテンツを地理的領域にバインドおよび制限します
Me.GeoMap.WorldRect = New Rectangle(-30, -40, 120, 80)
C# の場合:
// 0.05 の係数でパンレフト(西方向)します
this.GeoMap.WindowPositionHorizontal = this.GeoMap.WindowRect.X - 0.05;
// 0.05 の係数でパンライト(東方向)します
this.GeoMap.WindowPositionHorizontal = this.GeoMap.WindowRect.X + 0.05;
// 0.05 の係数でパンアップ(北方向)します
this.GeoMap.WindowPositionVertical = this.GeoMap.WindowRect.Y - 0.05;
// 0.05 の係数でパンダウン(南方向)します
this.GeoMap.WindowPositionVertical = this.GeoMap.WindowRect.Y + 0.05;
Visual Basic の場合:
' 0.05 の係数でパンレフト(西方向)します
Me.GeoMap.WindowPositionHorizontal = Me.GeoMap.WindowRect.X - 0.05
' 0.05 の係数でパンライト(東方向)します
Me.GeoMap.WindowPositionHorizontal = Me.GeoMap.WindowRect.X + 0.05
' 0.05 の係数でパンアップ(北方向)します
Me.GeoMap.WindowPositionVertical = Me.GeoMap.WindowRect.Y - 0.05
' 0.05 の係数でパンダウン(南方向)します
Me.GeoMap.WindowPositionVertical = Me.GeoMap.WindowRect.Y + 0.05
以下のトピックでは、このトピックに関連する情報を提供しています。