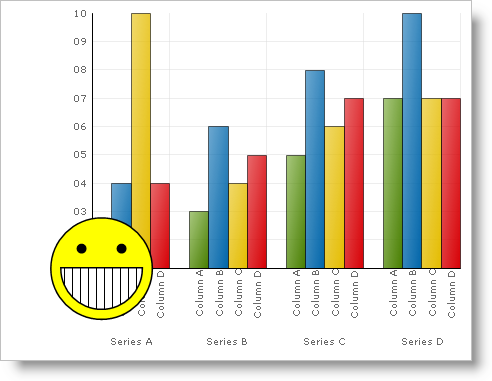
カスタム注釈を作成するには、 IAnnotation を実装するか Annotation を継承します。カスタム注釈を作成すると、これらのカスタム注釈のインスタンスをチャートの Annotations コレクションに追加できます。
Visual Basic の場合:
Me.UltraChart1.Annotations.Add(New MyAnnotation())
C# の場合:
this.ultraChart1.Annotations.Add(new MyAnnotation());
IAnnotation インタフェースは、Location という 1 つのプロパティだけで構成されます。このインタフェースを実装する場合、このプロパティをクラス内のプライベート フィールドに格納するだけで済みます。
IAnnotation インタフェースの重要部分は、 RenderAnnotation メソッドです。このメソッドには 2 つのオーバーロードがあります。1 つは、親のプリミティブを表す Primitive オブジェクトを受け取ります。もう 1 つのオーバーロードは Annotation を描画するポイントを表す Point オブジェクトを受け取ります。
これらの異なるオーバーロードがあるのは、 Location オブジェクトを使用して注釈をグラフに適合させる方法の違いによります。行または列(あるいはその両方)のワイルドカードを使用する場合、このメソッドは各グラフの注釈ごとに複数回呼び出すことができます。
親のプリミティブをパラメータとして持つ RenderAnnotation のオーバーロードの場合、親プリミティブから描画ポイントを取得する簡単な方法として、スタティックな Annotation. GetRenderPointFromParent メソッドを提供します。Annotation クラスをさらにカスタマイズする場合、親 プリミティブ に基づいて適切なポイントを取得するためのカスタム コードが必要になる可能性があります。この段階で、RenderAnnotation のもう一方のオーバーロードを呼び出すことができます。もちろん、これは必要条件でなく、プログラミングのガイドラインにすぎません。
注釈を描画するコードは、カスタム レイヤの FillSceneGraph メソッド内で使用されるコードと同じです。プリミティブを作成して SceneGraph に追加する必要があります。
以下は、カスタムの Annotation クラスの例です。これは、単純ですが、完成しています。
Visual Basic の場合:
Imports System Imports System.Drawing Imports System.Drawing.Drawing2D Imports Infragistics.UltraChart.Core Imports Infragistics.UltraChart.Core.Primitives Imports Infragistics.UltraChart.Resources.Appearance Imports Infragistics.UltraChart.Shared.Styles Public Class MyAnnotation Implements IAnnotation Public Sub RenderAnnotation(ByVal scene As SceneGraph, _ ByVal renderPoint As Point) Implements IAnnotation.RenderAnnotation Dim head As New Ellipse(renderPoint, 50) head.PE.Fill = Color.Yellow head.PE.StrokeWidth = 5 scene.Add(head) Dim mouth As New Wedge(renderPoint, 40, 0, 180) mouth.PE.Fill = Color.Black mouth.PE.FillStopColor = Color.White mouth.PE.ElementType = PaintElementType.Hatch mouth.PE.Hatch = FillHatchStyle.Vertical mouth.PE.StrokeWidth = 3 scene.Add(mouth) Dim leftEye As New Ellipse(New Point(renderPoint.X - 20, renderPoint.Y - 20), 5) Dim rightEye As New Ellipse(New Point(renderPoint.X + 20, renderPoint.Y - 20), 5) leftEye.PE.Fill = Color.Black rightEye.PE.Fill = Color.Black scene.Add(leftEye) scene.Add(rightEye) End Sub Public Sub RenderAnnotation(ByVal scene As SceneGraph, _ ByVal parent As Primitive) Implements IAnnotation.RenderAnnotation Dim renderPoint As Point = Annotation.GetRenderPointFromParent(parent) Me.RenderAnnotation(scene, renderPoint) End Sub Private _location As New Location() Public Property Location() As Location Implements IAnnotation.Location Get Return Me._location End Get Set(ByVal Value As Location) Me._location = Value End Set End Property End Class
C# の場合:
using System; using System.Drawing; using System.Drawing.Drawing2D; using Infragistics.UltraChart.Core; using Infragistics.UltraChart.Core.Primitives; using Infragistics.UltraChart.Resources.Appearance; using Infragistics.UltraChart.Shared.Styles; public class MyAnnotation : IAnnotation { public void RenderAnnotation(SceneGraph scene, Point renderPoint) { Ellipse head = new Ellipse(renderPoint, 50); head.PE.Fill = Color.Yellow; head.PE.StrokeWidth = 5; scene.Add(head); Wedge mouth = new Wedge(renderPoint, 40, 0, 180); mouth.PE.Fill = Color.Black; mouth.PE.FillStopColor = Color.White; mouth.PE.ElementType = PaintElementType.Hatch; mouth.PE.Hatch = FillHatchStyle.Vertical; mouth.PE.StrokeWidth = 3; scene.Add(mouth); Ellipse leftEye = new Ellipse(new Point(renderPoint.X - 20, renderPoint.Y - 20), 5); Ellipse rightEye = new Ellipse(new Point(renderPoint.X + 20, renderPoint.Y - 20), 5); leftEye.PE.Fill = Color.Black; rightEye.PE.Fill = Color.Black; scene.Add(leftEye); scene.Add(rightEye); } public void RenderAnnotation(SceneGraph scene, Primitive parent) { Point renderPoint = Annotation.GetRenderPointFromParent(parent); this.RenderAnnotation(scene, renderPoint); } private Location _location = new Location(); public Location Location { get { return this._location; } set { this._location = value; } } }