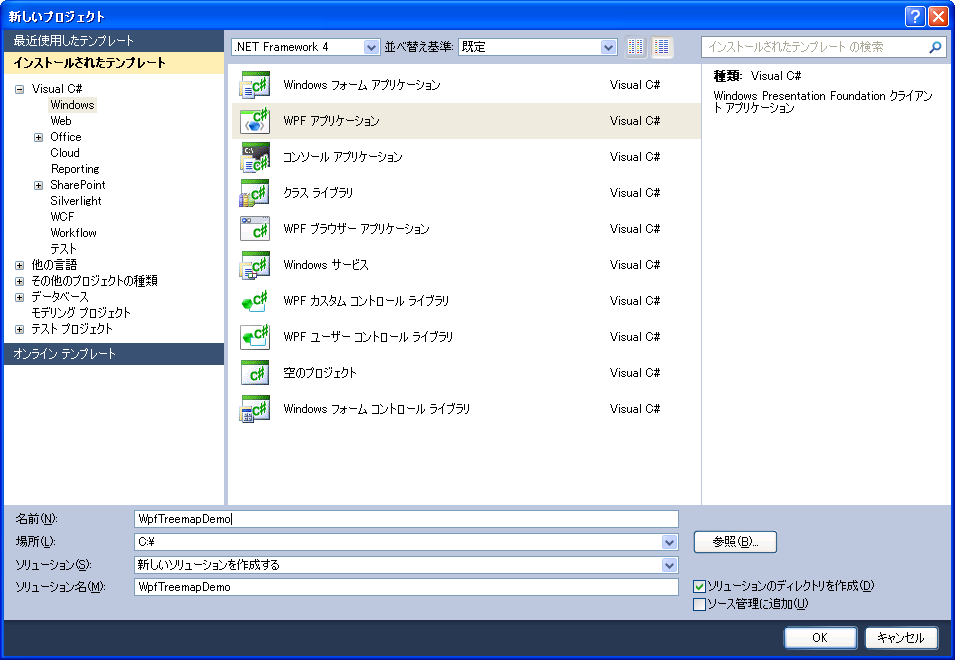
ここでは、xamTreemap コントロールを ADO.NET エンティティ データ モデル にバインドする方法を学習します。
WpfTreemapDemo という名前の新しい Windows Presentation Foundation アプリケーションを Visual Studio 2010 で作成します。
以下の NuGet パッケージをアプリケーションに追加します。
Infragistics.WPF.Treemap
NuGet フィードのセットアップと NuGet パッケージの追加の詳細については、NuGet フィード ドキュメントを参照してください。
プロジェクトを右クリックして、[追加] > [新しい項目] を選択します。
TreemapModel という名前の新しい ADO.NET エンティティ データ モデルを作成します。
データベースを選択します。このサンプルで、サンプル データベース AdventureWorks2008 に接続します。
必要なテーブルを選択します。この例では、ProductCategory、ProductSubcategory および Product で作業をします。
リンクされたこれらのテーブルは、xamTreemap コントロールに渡す階層的なデータ ソースになります。Visual Studio は使用するナビゲーション プロパティを作成します。
ProductCategory.ProductSubcategories
ProductSubcategory.Products
MainWindow.xaml を編集します:
XAML の場合:
<Window x:Class="WpfTreemapDemo.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:ig="http://schemas.infragistics.com/xaml" Loaded="MainWindow_Loaded" Title="MainWindow"> <Window.Resources> <!--xamTreemap コントロールのデータ ソース--> <CollectionViewSource x:Key="ProductCategoriesViewSource" /> </Window.Resources> <Grid DataContext="{StaticResource ProductCategoriesViewSource}"> <ig:XamTreemap x:Name="Treemap" ItemsSource="{Binding}"> <ig:XamTreemap.NodeBinders> <!--ProductCategory 項目のバインダー--> <ig:NodeBinder TextPath="Name" TargetTypeName="ProductCategory" ItemsSourcePath="ProductSubcategories" /> <!--ProductSubcategory 項目のバインダー--> <ig:NodeBinder TextPath="Name" TargetTypeName="ProductSubcategory" ItemsSourcePath="Products" /> <!--Product 項目のバインダー--> <ig:NodeBinder TextPath="Name" ValuePath="StandardCost" TargetTypeName="Product" /> </ig:XamTreemap.NodeBinders> </ig:XamTreemap> </Grid> </Window>
MainWindow.xaml のコード ビハインド ファイルで以下を追加します。
Visual Basic の場合:
Imports System.Data.Objects Class MainWindow Private Sub Window_Loaded _ (ByVal sender As System.Object, ByVal e As System.Windows.RoutedEventArgs) Dim adventureWorks2008Entities As New AdventureWorks2008Entities() 'MainWindow.xaml から CollectionViewSource リソースのインスタンスを取得します Dim productCategoriesViewSource As CollectionViewSource = DirectCast(Me.FindResource("ProductCategoriesViewSource"), CollectionViewSource) 'ProductCategory 項目の新しいクエリを作成します Dim productCategoriesQuery As ObjectQuery(Of WpfTreemapDemo.ProductCategory) = adventureWorks2008Entities.ProductCategories 'クエリを実行します productCategoriesViewSource.Source = productCategoriesQuery.Execute(MergeOption.AppendOnly) End Sub End Class
C# の場合:
using System.Data.Objects; using System.Windows; using System.Windows.Data; namespace WpfTreemapDemo { public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } void MainWindow_Loaded(object sender, RoutedEventArgs e) { AdventureWorks2008Entities adventureWorks2008Entities = new AdventureWorks2008Entities(); //MainWindow.xaml から CollectionViewSource リソースのインスタンスを取得します CollectionViewSource productCategoriesViewSource = (CollectionViewSource)(this.FindResource("ProductCategoriesViewSource")); //ProductCategory 項目の新しいクエリを作成します ObjectQuery<WpfTreemapDemo.ProductCategory> productCategoriesQuery = adventureWorks2008Entities.ProductCategories; //クエリを実行します productCategoriesViewSource.Source = productCategoriesQuery.Execute(MergeOption.AppendOnly); } } }
アプリケーションを実行します。
関連トピック